This guy walked on a loan of 45k BEWARE!
Jp currently resides at 1625 Bear Mountain Driv, Boulder, CO 80305-6836. There is one company at this address that we know about — Jp O'brien. Nine persons linked to this address. Their name are Allen J Kandell, Carol A Kandell, and seven others. Jp is the owner of phone number (303) 324-9177 (New Cingular Wireless Pcs, LLC). There is a chance that the phone number (303) 324-9177 is shared by Jennifer A O'brien, Jean Obrien
- 1625 Bear Mountain Dr, Boulder, CO 80305
- Single Family, Attached Garage, 2 spaces, 575 sqft garage
- Four bedrooms, 3.5 bathrooms
- Lot Size - 0.46 acres, Floor Size - 3,900 sqft
- Parcel ID# 157707410001
- Last Sale Nov 2007 - Price $1,000,000
- County: Boulder County
- Neighborhood: Devil's Thumb - Rolling Hill
- FIPS: 80130125103029
- Possible connections via main address - Allen J Kandell, Carol A Kandell
- Latitude, Longitude: 39.971683, -105.265126
- (303) 324-9177
- Possible connections via phone numbers - Jennifer A O'brien, Jean Obrien
https://www.denverpost.com/2016/06/19/black-lab-sports-pro-athletes-business-boulder/
https://www.blacklabx.com/investors
https://rocketreach.co/jean-paul-obrien-email_40795910
https://www.linkedin.com/in/jeanpaulobrien
https://www.crunchbase.com/person/jp-o-brien
https://whatgotyouthere.com/portfolio/jp-obrien-seandelaney/
https://x.com/jp_obrien
https://www.blacklabx.com/team
https://www.flywheelproject.com/team
https://www.youtube.com/watch?v=mBPMrgjAsb0
https://www.linkedin.com/in/jenniferobrien01/
Jean-Paul O’Brien
CEO
O’Brien, Founder and CEO, is a serial entrepreneur, investor, and community builder. O'Brien is on a mission to inspire, activate, and hyper-enable Thinker Doers (scientists, entrepreneurs, investors, elite athletes, and creatives) to propel humanity forward. Black Lab builds and invests in HumanTech ventures and human potential nodes, with themes centered around Longevity, Consciousness, Community, and Environment.

Aaron Beach
COO
Beach, Co-Founder and Head of Operations, brings his passion, experience, and expertise in human resources and operations. Beach’s strengths include creating native work cultures specifically centered on the people who lead to its success, as well as fostering inspiring and empowering work environments for business growth.
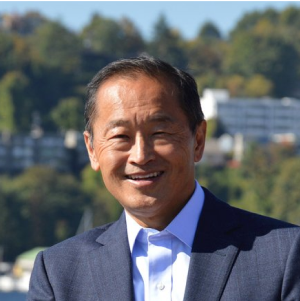
Chris Ishii

Jennifer O'Brien
MANAging director
Ms. O’Brien, Managing Director,
Ms. O’Brien, Managing Director, has a long history of using technology to solve business problems and deliver tangible top- and bottom- line impact. She possesses a growth mindset and well-rounded background that spans complex, global program delivery, account management, and product line development, as well as experience working with leaders across the healthcare and life sciences, high-tech, consumer products, and retail sectors. Prior to Black Lab X, Ms. O’Brien was a Partner in West Monroe’s M&A practice where she worked with Private Equity firms to drive strategic value in their portfolio, including delivering technology-driven value creation projects for portfolio companies, providing sell-side advisory services, and conducting technology due diligences.